Custom Themes with the Partner API
You may wish to customize the onboarding experience for your customers. We've provided a themes
endpoint that allows you to customize the brand name, logo, and a few other elements within the onboarding flow.
Once you have created your theme, you can apply it by specifying the theme_id="your theme ID"
as part of the direct login URL you generate for a ShipEngine user account.
Customizable Elements
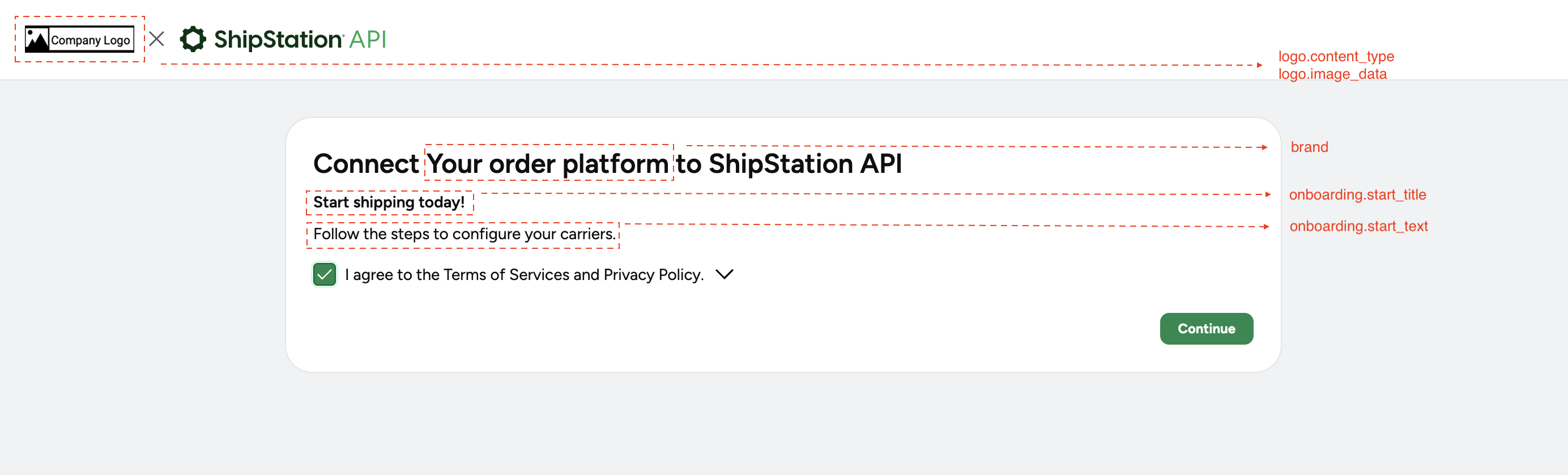
- Logo image (this image will persist throughout your customer's portal experience)
- Brand name
- Start title
- Start text
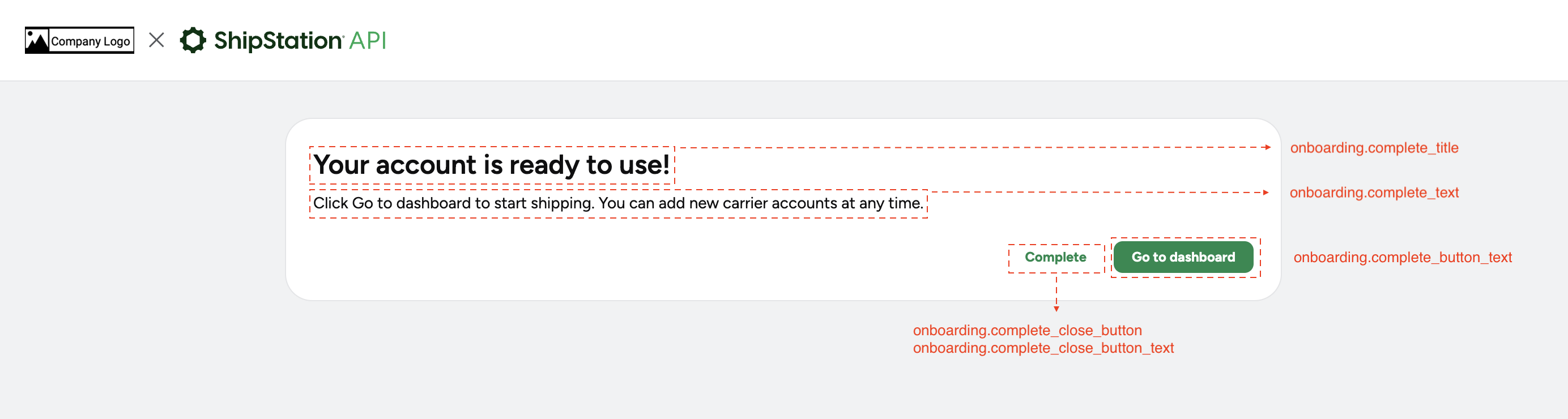
- Complete title
- Complete text
- Complete button text
- Close button
- Close button text
Properties
The following properties are associated with the themes
endpoint:
Property | Type | Description |
---|---|---|
name | string | Required The name of the theme Max 50 characters |
logo.content_type | image/jpeg image/png | Image content type |
logo.image_data | string | base64 encoded |
brand | string | Required Brand name that appears on the Start screen |
return_url | string | URL to return to upon logout Max 1000 characters |
onboarding.start_title | string | Max 50 characters |
onboarding.start_text | string | Max 1000 characters |
onboarding.complete_title | string | Max 50 characters |
onboarding.complete_text | string | Max 1000 characters |
onboarding.complete_button_text | string | Max 50 characters |
onboarding.complete_close_button | boolean | Default = false Determines whether to display the close button or not (see About section below for behavior details) |
onboarding.complete_close_button_text | string | Max 50 characters |
theme_id | string | uuid |
created_at | string | date-time |
modified_at | string | date-time |
About the Close Button Behavior
The button will not close the onboarding window by itself, due to certain browser restrictions. To close the window, we'll post a message (shipengine.onboarding.close.clicked) through the window.opener
. The parent application must listen to that message and execute the close action.
For example, when the user clicks the close button, we will execute the following code:
Your parent application (that initially opened the dashboard) should have code similar to this:
Create a Theme
Use the themes
endpoint to create a new theme. You can create a maximum of 10 themes per partner.
Create Theme Example Request
POST /v1/partners/themes
Create Theme Example Response
Edit a Theme
When you create a theme, the response will include a theme_id
. You can then use the ID to make edits to your existing theme(s).
Edit Theme Example Request
PATCH /v1/partners/themes/{themeID}
Edit Theme Example Response
Delete a Theme
You can also delete any theme by its theme_id
.
Delete Theme Example Request
DELETE /v1/partners/themes/{themeId}
Delete Theme Example Response
GET Themes
Lastly, you can get the themes you've created by ID...
GET /v1/partners/themes/{themeId}
Or, get all the themes you've created...
GET /v1/partners/themes